mirror of
https://github.com/MathiasLui/CSGO-Projects.git
synced 2025-07-11 21:01:17 +00:00
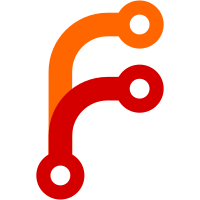
* Adjusted help window * Moved all settings that get saved in a file into a single window in the Edit menu * Add NAV area support and Z coordinate * Add weapon and NAV info boxes * Changed copying coordinates to include the setpos_exact command for ease of use * Settings are now saved and loaded * Add map scale factor override and X/Y offset * Removed ability to hide right click/left click circles * Add various summaries * Add OverwriteMapping class as an overwrite object for a map, that gets loaded from the settings and applied to each map when loading them * Add Associated area to map points and Z height
48 lines
1.5 KiB
C#
48 lines
1.5 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Linq;
|
|
using System.Text;
|
|
using System.Threading.Tasks;
|
|
using System.Xml.Serialization;
|
|
|
|
namespace Damage_Calculator
|
|
{
|
|
public static class Globals
|
|
{
|
|
public static Settings Settings { get; set; } = new Settings();
|
|
|
|
public static void LoadSettings()
|
|
{
|
|
// Get path
|
|
string pathToFile = System.IO.Path.Combine(MainWindow.FilesPath, Settings.SettingsFileName);
|
|
|
|
if (!System.IO.File.Exists(pathToFile))
|
|
{
|
|
Globals.SaveSettings();
|
|
return;
|
|
}
|
|
|
|
XmlSerializer serializer = new XmlSerializer(typeof(Settings));
|
|
using (var fs = new System.IO.FileStream(pathToFile, System.IO.FileMode.Open))
|
|
{
|
|
Globals.Settings = (Settings)serializer.Deserialize(fs);
|
|
}
|
|
}
|
|
|
|
public static void SaveSettings()
|
|
{
|
|
// Get path
|
|
string pathToFile = System.IO.Path.Combine(MainWindow.FilesPath, Settings.SettingsFileName);
|
|
|
|
if (!System.IO.Directory.Exists(MainWindow.FilesPath))
|
|
// Make sure the folder exists before attempting to save the file
|
|
System.IO.Directory.CreateDirectory(MainWindow.FilesPath);
|
|
|
|
XmlSerializer serializer = new XmlSerializer(typeof(Settings));
|
|
using (var fs = new System.IO.FileStream(pathToFile, System.IO.FileMode.Create))
|
|
{
|
|
serializer.Serialize(fs, Globals.Settings);
|
|
}
|
|
}
|
|
}
|
|
}
|