mirror of
https://ceregatti.org/git/daniel/dayzdockerserver.git
synced 2025-07-12 04:11:18 +00:00
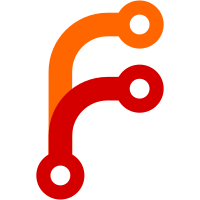
Use node js module syntax. Make dev easier by not starting the web UI from docker startup.
136 lines
4.4 KiB
JavaScript
136 lines
4.4 KiB
JavaScript
const template = `
|
|
<div class="container-fluid">
|
|
<div class="row jumbotron darkgrey">
|
|
<div class="col-10">
|
|
<h1>DayZ Docker Server</h1>
|
|
</div>
|
|
<div class="col-2">
|
|
<div>
|
|
Server files installed: {{ installed }}
|
|
</div>
|
|
<div>
|
|
Version: {{ version }}
|
|
</div>
|
|
</div>
|
|
</div>
|
|
<div
|
|
v-if="fetchError != ''"
|
|
class="row jumbotron text-center alert alert-danger"
|
|
>
|
|
{{ fetchError }}
|
|
</div>
|
|
<div class="row jumbotron darkgrey">
|
|
<div class="col-3">
|
|
<h2 class="text-center">Mods</h2>
|
|
<table>
|
|
<tr>
|
|
<th>Steam Link</th>
|
|
<th>Mod Info</th>
|
|
</tr>
|
|
<template
|
|
v-for="mod in mods"
|
|
:key="index"
|
|
>
|
|
<tr>
|
|
<td>
|
|
<a
|
|
target="_blank"
|
|
:href="'https://steamcommunity.com/sharedfiles/filedetails/?id=' + mod.id"
|
|
>
|
|
{{ mod.id }}
|
|
</a>
|
|
</td>
|
|
<td>
|
|
<a class="simulink" @click="getModInfo(mod.id)">{{ mod.name }}</a>
|
|
</td>
|
|
</tr>
|
|
</template>
|
|
</table>
|
|
</div>
|
|
<div class="col-9 modInfo" v-if="modInfo != ''">
|
|
<div class="text-center col-2">
|
|
<h2>{{ modInfo.name }}</h2>
|
|
</div>
|
|
<div class="row">
|
|
<div class="col-2">
|
|
<div>
|
|
ID: {{ modInfo.id }}
|
|
</div>
|
|
<div>
|
|
Size: {{ modInfo.size.toLocaleString("en-US") }}
|
|
</div>
|
|
<div v-if="modInfo.customXML.length > 0">
|
|
Custom XML files:
|
|
<ul>
|
|
<li v-for="info in modInfo.customXML">
|
|
<a class="simulink" @click="getXMLInfo(modInfo.id,info.name)">{{ info.name }}</a>
|
|
</li>
|
|
</ul>
|
|
</div>
|
|
</div>
|
|
<div class="col-10">
|
|
<textarea cols="120" rows="15" v-if="this.XMLInfo != ''">{{ this.XMLInfo }}</textarea>
|
|
</div>
|
|
</div>
|
|
</div>
|
|
</div>
|
|
</div>
|
|
</template>
|
|
`
|
|
|
|
export default {
|
|
name: 'DazDockerServer',
|
|
template: template,
|
|
data() {
|
|
return {
|
|
fetchError: "",
|
|
installed: false,
|
|
mods: [],
|
|
modInfo: "",
|
|
version: "Unknown",
|
|
XMLInfo: "",
|
|
}
|
|
},
|
|
methods: {
|
|
getModInfo(modId) {
|
|
fetch('/mod/' + modId)
|
|
.then(response => response.json())
|
|
.then(response => {
|
|
this.modInfo = response
|
|
this.XMLInfo = ""
|
|
})
|
|
.catch((error) => {
|
|
console.error(error)
|
|
this.fetchError = error.message
|
|
})
|
|
},
|
|
getXMLInfo(modId, file) {
|
|
fetch('/mod/' + modId + '/' + file)
|
|
.then(response => response.text())
|
|
.then(response => {
|
|
this.XMLInfo = response
|
|
})
|
|
.catch((error) => {
|
|
console.error(error)
|
|
this.fetchError = error.message
|
|
})
|
|
}
|
|
},
|
|
mounted() {
|
|
// Get the data
|
|
fetch('/status')
|
|
.then(response => response.json())
|
|
.then(response => {
|
|
this.installed = response.installed
|
|
this.version = response.version
|
|
this.mods = response.mods
|
|
if(response.error) {
|
|
this.fetchError = response.error
|
|
}
|
|
})
|
|
.catch((error) => {
|
|
console.error(error)
|
|
this.fetchError = error.message
|
|
})
|
|
}
|
|
}
|