mirror of
https://ceregatti.org/git/daniel/dayzdockerserver.git
synced 2025-06-23 20:41:18 +00:00
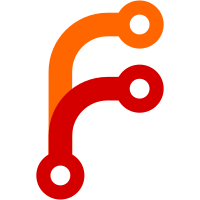
Renamed main container to web. Added the STEAMAPIKEY environment variable placeholder, as this is necessary when searching for mods. Refactor locations of scripts and paths. Rename start scripts to be more consistent with their new locations. Run the web server under nodemon to prevent manual restarting.
135 lines
3.4 KiB
Bash
Executable file
135 lines
3.4 KiB
Bash
Executable file
#!/usr/bin/env bash
|
|
|
|
set -eEa
|
|
|
|
# If you want/need the server and rcon ports to be different, set them here.
|
|
# The steam query port is set in serverDZ.cfg.
|
|
|
|
# Server port
|
|
port=2302
|
|
rcon_port=2303
|
|
|
|
# Don't change anything else.
|
|
|
|
# Colors
|
|
default="\e[0m"
|
|
red="\e[31m"
|
|
green="\e[32m"
|
|
yellow="\e[93m"
|
|
lightblue="\e[94m"
|
|
blue="\e[34m"
|
|
magenta="\e[35m"
|
|
cyan="\e[36m"
|
|
|
|
# DayZ release server Steam app ID. USE ONE OR THE OTHER!!
|
|
# Presumably once the Linux server is released, the binaries will come from this ID.
|
|
# But more importantly, if we have a release-compatible binary, the base files must be installed from this id,
|
|
# even if the server binary and accompanying shared object don't come from it.
|
|
#release_server_appid=223350
|
|
# Without a release binary, we must use the experimental server app id for everything.
|
|
release_server_appid=1042420
|
|
|
|
# DayZ release client SteamID. This is for mods, as only the release client has them.
|
|
release_client_appid=221100
|
|
|
|
# Common container base directories
|
|
FILES="/files"
|
|
SERVER_FILES="/serverfiles"
|
|
|
|
# Used to check if dayZ is installed
|
|
SERVER_INSTALL_FILE="${SERVER_FILES}/DayZServer"
|
|
|
|
# Steam files
|
|
STEAM_LOGIN="${HOME}/steamlogin"
|
|
STEAMCMD=steamcmd
|
|
|
|
# Workshop files (mods)
|
|
WORKSHOP_DIR="${SERVER_FILES}/steamapps/workshop/content/${release_client_appid}"
|
|
|
|
# Other stuff
|
|
YES="${green}yes${default}"
|
|
NO="${red}no${default}"
|
|
|
|
# Functions
|
|
|
|
# Convenience function
|
|
prompt_yn(){
|
|
echo -n "${1} (y|N) " >&2
|
|
read -s -n 1 a
|
|
a=$(echo ${a} | tr A-Z a-z)
|
|
echo
|
|
if [[ "${a}" = "y" ]]
|
|
then
|
|
return 0
|
|
else
|
|
return 1
|
|
fi
|
|
}
|
|
|
|
check_mod_install(){
|
|
# See if this mod id exists in files/mods, and offer to install other server side files if an install.sh is found
|
|
if [ -f ${FILES}/mods/${1}/${2}.sh ]
|
|
then
|
|
echo "An ${2}.sh was found for mod id ${1}. Running..."
|
|
${FILES}/mods/${1}/${2}.sh
|
|
fi
|
|
# A generic map install script. Presumes a git repo as the source
|
|
if [ -f ${FILES}/mods/${1}/install.env ]
|
|
then
|
|
echo "An ${2}.env was found for mod id ${1}. Performing ${2}..."
|
|
source ${FILES}/mods/${1}/install.env
|
|
${FILES}/mods/install.sh ${1} ${2}
|
|
fi
|
|
}
|
|
|
|
get_mod_id_by_index2(){
|
|
# If we were passed a valid mod id, just return it
|
|
if [ -d "${WORKSHOP_DIR}/${1}" ]
|
|
then
|
|
echo -n ${1}
|
|
return
|
|
fi
|
|
X=1
|
|
# Loop over mods
|
|
for dir in $(ls -tr ${WORKSHOP_DIR})
|
|
do
|
|
ID=${dir}
|
|
if [[ ${X} = ${1} ]]
|
|
then
|
|
echo -n ${ID}
|
|
return
|
|
fi
|
|
X=$((X+1))
|
|
done
|
|
}
|
|
|
|
# Get mod name by ID or index
|
|
get_mod_name(){
|
|
ID=$(get_mod_id_by_index2 ${1})
|
|
if ! [ -d "${WORKSHOP_DIR}/${ID}" ]
|
|
then
|
|
echo "Mod ID ${1} doesn't exist" >&2
|
|
exit 1
|
|
fi
|
|
NAME=$(grep name ${WORKSHOP_DIR}/${ID}/meta.cpp | cut -d '"' -f2 | sed -r 's/\s+//g')
|
|
echo -n ${NAME}
|
|
}
|
|
|
|
# List mods
|
|
list(){
|
|
X=1
|
|
C="${green}"
|
|
spaces=" "
|
|
echo "Installed mods:"
|
|
echo -e " ID Name URL Size"
|
|
echo "------------------------------------------------------------------------------------------------------------------------"
|
|
for dir in $(ls -tr ${WORKSHOP_DIR})
|
|
do
|
|
ID=${dir}
|
|
NAME=$(grep name "${WORKSHOP_DIR}/${dir}/meta.cpp" | cut -d '"' -f2 | sed -r 's/\s+//g')
|
|
SIZE=$(du -sh "${WORKSHOP_DIR}/${dir}" | awk '{print $1}')
|
|
printf "${C}%.3d %s %.30s %s https://steamcommunity.com/sharedfiles/filedetails/?id=%s %s${default}\n" ${X} ${ID} "${NAME}" "${spaces:${#NAME}+1}" ${ID} ${SIZE}
|
|
X=$((X+1))
|
|
done
|
|
echo
|
|
}
|